What is Pydantic?
Pydantic is a Python package that can offer simple data validation and manipulation. It was developed to improve the data validation process for developers. Indeed, Pydantic is an API for defining and validating data that is flexible and easy to use, and it integrates seamlessly with Python's data structures. Developers can specify the Pydantic data validation rules and the data. The library will then automatically validate incoming data and raise errors if any rules are unmet. It makes ensuring project data is consistent and complies with standards easier.
How does Pydantic work?
Pydantic functions by letting programmers use Python classes to define data models. These classes can contain type hints, default values, and validation rules. They derive from the Pydantic BaseModel class. Pydantic Python uses the data model to verify incoming data and ensure it satisfies the specified requirements when received.
Pydantic validates the data by comparing each field to the type hints and validation rules set in the data model. Pydantic validator raises an error message and ends the validation process if the data does not match the requirements. Pydantic is responsible for creating an instance of the data model and loads the incoming data into it. After that, it returns the data to the user if data validation is failed.
To handle a variety of data validation scenarios, Pydantic also offers advanced features like field aliasing, custom validation functions, and support for nested data models. Pydantic also facilitates serialization and deserialization, allowing data conversion to and from Python data structures, JSON, and other formats.
How to use Pydantic to validate data?
Using Pydantic to validate data is a straightforward process. You can define a Pydantic model and then use that model to validate input data. Here is the Pydantic example to validate data:
The above example defines a User model with fields for age, password, and username. A custom validation rule that verifies the password field has at least eight characters in length is added using the validator decorator. The User model receives the incoming data, and Pydantic handles the validation automatically. The validation process ends, and an error is raised if the data is invalid.
You must note that the data structure is defined by Pydantic using type hints; the expected data types for the age, password, and username fields are indicated by the str and int type hints, respectively. Pydantic will automatically verify that the incoming data satisfies these requirements and raise errors if any data types are incorrect.

Benefits of using Pydantic
Pydantic offers several benefits when working with structured data, data validation, and parsing. Here are some of the key advantages of using Pydantic in your Python projects:
Data validation
Pydantic simplifies the data validation process. It can check input data automatically conforms to the specified data models and their constraints. It ensures the integrity and consistency of your data, which can reduce the risk of unexpected errors.
Type safety
Pydantic leverages Python's type hinting system. You can improve code readability by declaring data types using type annotations. It can also help developers to understand the expected data structures.
Automatic data conversion
Pydantic can parse and convert input data into Python objects, saving you from writing custom data conversion code. It handles tasks like converting strings to integers, dates, or custom data types and streamlining data processing.
Error handling
You will receive detailed and informative error messages if validation fails. It makes it easier to pinpoint issues in your input data and respond appropriately, whether by informing users of invalid input or logging errors for debugging.
Documentation
Pydantic use of type annotations serves as a form of self-documentation. Developers can easily understand the expected data structures and constraints by examining the Pydantic model classes.
Optional fields and default values
Pydantic allows you to define optional fields and specify default values for fields. This flexibility makes it easy to work with data where some fields are not always present or should have fallback values.
Custom validation logic
Pydantic enables you to implement custom validation logic within your model classes. You can define methods to perform complex checks on the input data and ensure it meets your application's specific requirements.
Integration with web frameworks
Pydantic is commonly used with web frameworks like FastAPI, where it simplifies handling request and response data. By defining Pydantic models for your API endpoints, you can automatically validate incoming requests and generate well-documented API documentation.
Cleaner code
With the help of Pydantic, you can write cleaner and more readable code. Data validation and conversion logic are encapsulated within your models that reduce the repetition and error-prone validation code.
Testing
Pydantic models makes it easier to write unit tests for your code. You can create instances of your models with test data and verify that they behave as expected.
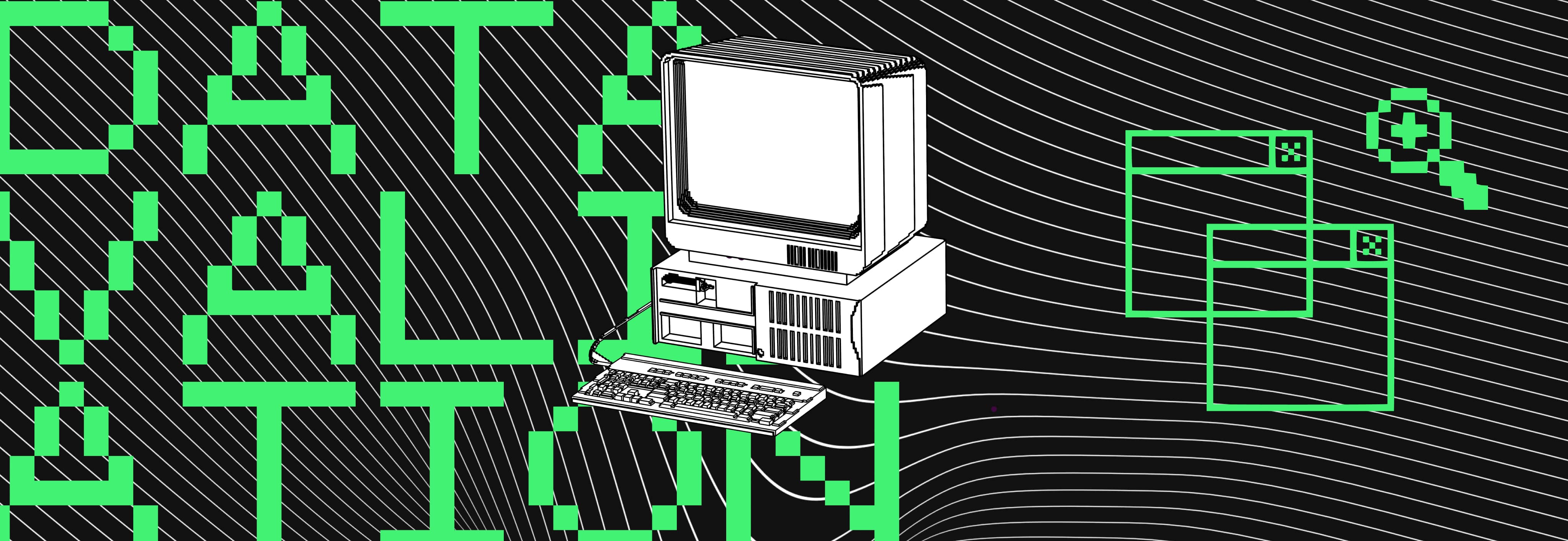
Advanced Pydantic features
Pydantic is a versatile Python library known for its data validation and parsing capabilities. Beyond the fundamental features, Pydantic offers several advanced features that give developers even more flexibility and power. These advanced features enhance the library's utility in various application domains. Here are some of the advanced features of Pydantic:
Data parsing and serialization
Pydantic is primarily used for data validation and handles data parsing and serialization. It helps build web applications and APIs where you must convert data between formats like JSON, YAML, or database records. Indeed, Pydantic can parse incoming data into Pydantic models automatically.
Custom validation methods
Pydantic allows you to define custom validation methods within your model classes. These methods can perform complex checks on the input data and help you implement custom validation logic tailored to your specific application requirements. This feature is especially valuable when your data validation needs to go beyond simple type checks.
Validation of nested models
Pydantic supports the validation of nested models, which means you can define complex data structures by nesting Pydantic models within each other. This feature is helpful when dealing with hierarchical or deeply nested data, such as configurations with multiple levels of nested options.
Data classes integration
Pydantic can be used in conjunction with Python's data classes. Pydantic data class provides a concise way to define a class for storing data without boilerplate code. When combined with Pydantic, you get the benefits of data classes along with Pydantic data validation and parsing features.
Field aliases
Pydantic allows you to specify field aliases, which are alternative names for fields in your data model. It is useful when your data source uses different field names than your model’s. Pydantic can also map the input data to the correct fields in your model by defining aliases.
Custom error messages
You can customize error messages for your validation rules. It helps provide more user-friendly error messages when validation fails, improving overall user experience and making error reporting more informative.
Constraints
Pydantic supports a wide range of constraints. These constraints include min and max values, regular expressions, and more. You can define constraints on your fields to ensure data meets specific requirements beyond just data types.
Dependent fields
Pydantic allows you to create dependent fields where the value of one field depends on the value of another. This feature is pretty useful when you need to enforce relationships between fields in your data model.
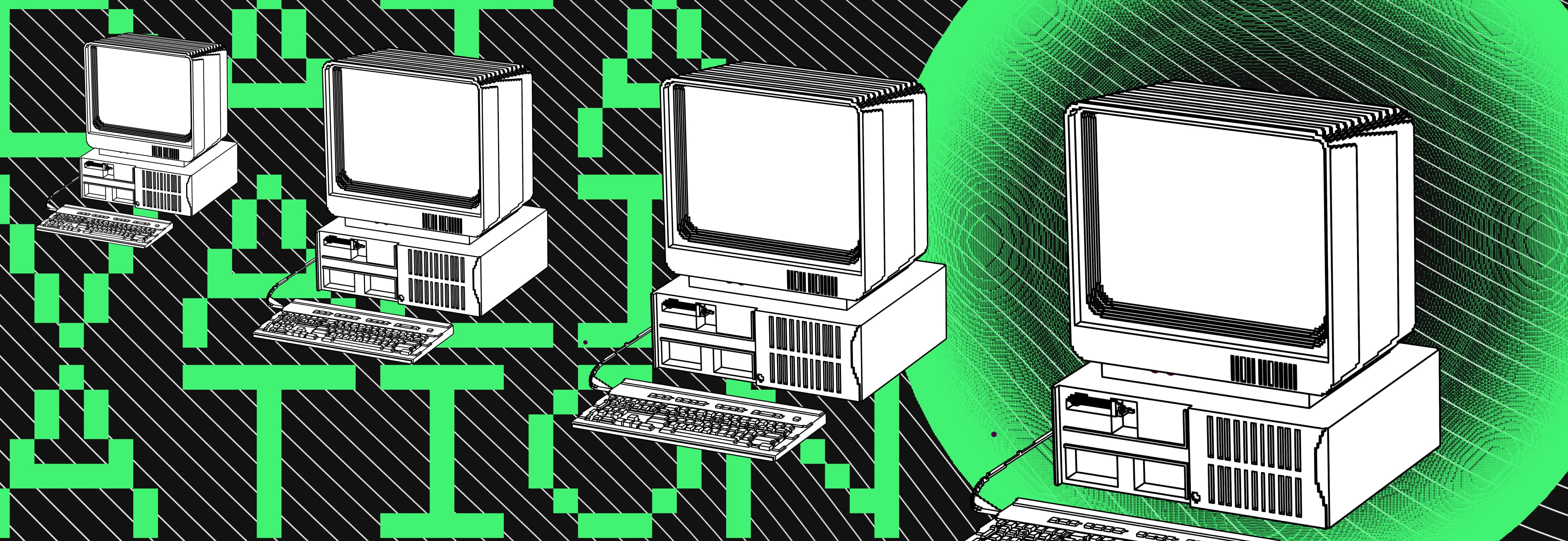
Pydantic FAQ
What distinguishes Pydantic from other validation libraries?
Pydantic is created explicitly for Python 3.7+ and uses type annotations to define the expected data structure, resulting in more readable and concise code. Additionally, it supports data serialization and deserialization and offers automatic data validation.
How do I add custom validation logic in Pydantic
The validator decorator allows developers to define extra validation logic performed when incoming data is validated, allowing them to add custom validation functions.
Does Pydantic support nested data structures?
Yes, Pydantic enables the use of nested data models and makes it simple for developers to define complex data structures
Final thoughts
In short, Pydantic is a powerful Python library that makes modeling, parsing, and data validation easier. Developers have access to a versatile tool for handling complex data structures thanks to its sophisticated features, which include support for nested models, data serialization, and custom validation techniques. Pydantic improves data validation and user experience by integrating easily with Python's type hinting system and enabling custom error messages, field aliases, and constraints.
Moreover, Pydantic Python can help develop web applications and APIs and work with configuration data because of its adaptability, extensibility, and data class compatibility. Pydantic enables developers to effectively handle structured data in a variety of application domains while also promoting data integrity and code clarity.